Applied Design Patterns with Java
Structural :: Flyweight (195) {C ch 14}
Applicability
The Flyweight pattern's effectiveness depends heavily on how and where it's used. Apply the
Flyweight
pattern when ALL
of the following are true:
- An application
uses a large number of objects;
- Storage costs are high because of the sheer quantity
of objects;
- Most object states can be made extrinsic;
- Many groups of objects may be replaced by relatively
few shared objects once extrinsic state is removed;
- The application doesn't depend on object identity. Since
Flyweight objects may be shared, identity tests will return true for conceptually distinct objects.
Structure
The Flyweight pattern has this general organization:
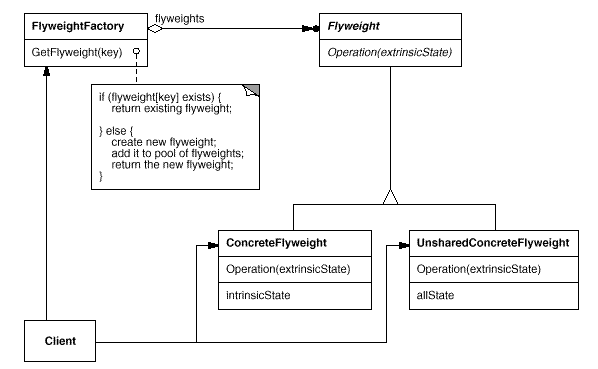
The following object diagram shows how Flyweights are shared:
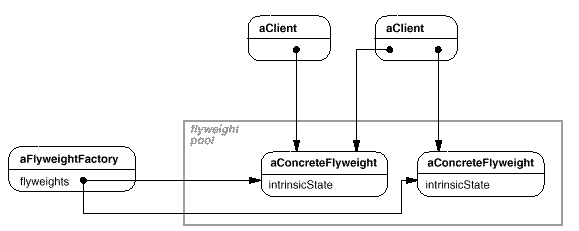
Participants
- Flyweight declares an interface through which Flyweights can receive and act on extrinsic state.
- ConcreteFlyweight (Character) implements the Flyweight interface and adds storage for intrinsic state, if any. A ConcreteFlyweight
object must be sharable. Any state it stores must be
intrinsic; that is, it must be independent of the ConcreteFlyweight
object's context.
- UnsharedConcreteFlyweight (Row, Column)
not all Flyweight subclasses need
to be shared. The Flyweight interface enables sharing; it doesn't enforce it. It's common for UnsharedConcreteFlyweight
objects to have ConcreteFlyweight objects as children at some level in the Flyweight object structure
(as the Row and Column classes have).
- FlyweightFactory
creates and manages flyweight objects, and ensures that Flyweights are shared properly. When a client requests a Flyweight, the FlyweightFactory object
supplies an existing instance or creates one, if none exists.
- Client maintains
a reference to Flyweight(s), and computes or stores the extrinsic state of Flyweight(s).
Catalog Structural Prev Next