Applied Design Patterns with Java
Structural :: Bridge (151) {C ch 10}
Applicability
Use the Bridge pattern when:
- to avoid a permanent binding between an abstraction
and its implementation. This might be the case when the implementation must be selected or switched at run-time;
- both the abstractions and their implementations should
be extensible by subclassing. The Bridge pattern allows combining different abstractions and implementations
and extending them independently;
- changes in the implementation of an abstraction should
have no impact on clients; their code should not have to be recompiled;
- there is a need to hide the implementation of an abstraction
completely from clients;
- a proliferation of classes exists, and there is a need
for splitting an object into two parts (Rumbaugh's "nested generalizations");
- to share an implementation among multiple objects, and
this fact should be hidden from the client.
Structure
The Bridge pattern has this general organization:
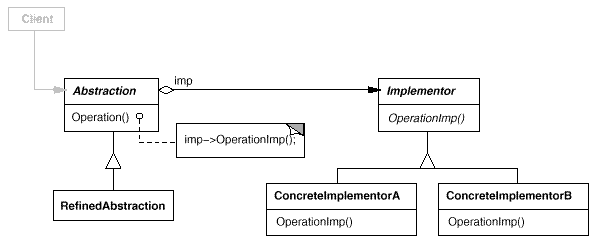
Participants
- Abstraction (Window) defines the abstraction's interface and maintains a reference to an object of
type Implementor.
- RefinedAbstraction (IconWindow) extends the interface defined by Abstraction.
- Implementor (WindowImp) defines the interface for implementation classes. This interface doesn't have
to correspond to Abstraction's interface; in fact the interfaces can be quite different. The Implementor interface
provides primitive operations, and Abstraction defines higher-level operations based on these primitives.
- ConcreteImplementor (XWindowImp, PMWindowImp)
implements the Implementor interface and defines its concrete implementation.
Catalog Structural Prev Next