Applied Design Patterns with Java
Behavioral :: Template Method (325) {C ch 25}
Example - Java :: Patterns\Behavioral\Template_Method
Pattern Concept: to define the skeleton of an algorithm in
an operation, deferring some steps to subclasses. Template
Method lets subclasses redefine certain steps of an algorithm
without changing the algorithm's structure. This is
a simple, but very common and widely used pattern. Essentially, an algorithm is defined in an abstract class, and
the finishing implementation details are left to subclasses. Another core idea is that there are some basic parts
of a class that can be "factored" out and put in a base class, so that they do not need to be repeated
in several subclasses.
There are four basic kinds of base class methods that can
be used in derived classes:
- Complete methods that carry out some basic function that
all of the subclasses will reuse. These are referred to as Concrete methods.
- Methods that are not filled in at all and must be implemented
in derived classes. These are referred to as Abstract methods.
- Methods that contain a default implementation of some
operation, but which may be overriden in derived classes. These are referred to as Hook methods.
- A class may contain methods that call any combination
of Concrete, Abstract, and Hook
methods. These are the Design Pattern's
Template Methods.
Cooper's Template
Method example again works with drawing in a graphic context.
The Template Method
algorithm is draw2ndLine(), and it is used in Triangle subclasses for the drawing of various types of triangles:
standard, isosceles, and right triangles.
Example - UML : TriangleDrawing
Here is Cooper's Class Diagram for the application using the Template Method
pattern, followed by the Rose equivalent:
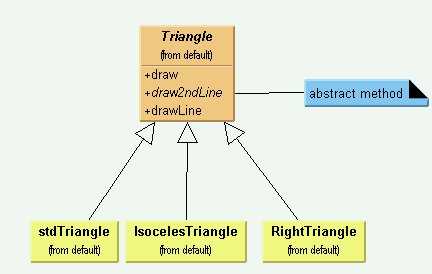
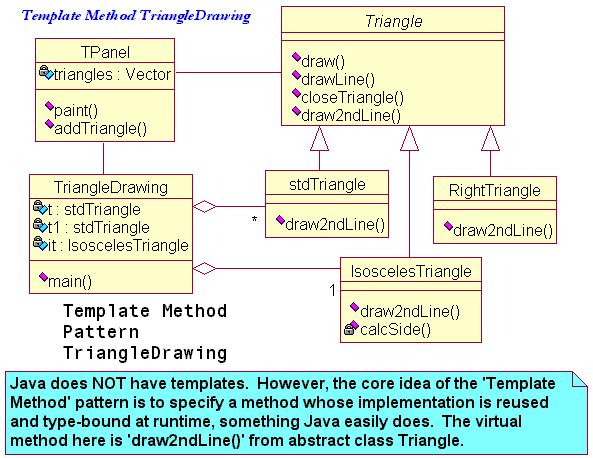
The example Java program is called 'TriangleDrawing'.
The UML diagram is above, and the list of Java files is
below:
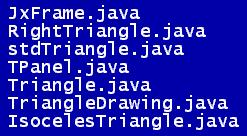
Issues and consequences of the Template Method
pattern include:
- The Template
Method pattern allows the base class to define
only some of the methods that will be used; the rest can be implemented in the derived classes.
- There might be methods in the base class that call a sequence
of methods, some implemented in the base class, and some implemented in the derived class. The base class defines
the outline of the method, but many details can be left to the derived classes.
- Some base class abstract methods might need to be overridden
in the derived classes, or there might be stub functions in the base class that need to be overriden in the placeholder
derived classes. If these placeholders are called from ANOTHER method
in the base class, then the overridden methods are Hook methods.
- At the point a Template
Method invocation occurs at runtime, Java ALWAYS looks FIRST
at the most local classes for the method implementation. If the method is not defined or overridden locally, Java
will then start moving up the inheritance tree, until it finds the appropriate method - all the way back to the
base class if necessary.
Catalog Behavioral Prev Next